一文搞定 Python 读取文件的全部知识

使用上下文管理器打开文件
Python 中的文件读取模式
读取 text 文件
读取 CSV 文件
读取 JSON 文件

打开文件
open()
函数接受两个基本参数:文件名和模式open()
函数提供了几种不同的模式,我们将在后面逐一讨论f = open('zen_of_python.txt', 'r')
print(f.read())
f.close()
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
...
open()
函数以只读模式打开文本文件,这允许我们从文件中获取信息而不能更改它。在第一行,open()
函数的输出被赋值给一个代表文本文件的对象f
,在第二行中,我们使用read()
方法读取整个文件并打印其内容,close()
方法在最后一行关闭文件。需要注意,我们必须始终在处理完打开的文件后关闭它们以释放我们的计算机资源并避免引发异常with
上下文管理器来确保程序在文件关闭后释放使用的资源,即使发生异常也是如此:with open('zen_of_python.txt') as f:
print(f.read())
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
...
with
语句创建了一个上下文,并绑定到变量 f
,所有文件对象方法都可以通过该变量访问文件对象。read()
方法在第二行读取整个文件,然后使用 print()
函数输出文件内容f
变量,但是该文件是已关闭状态。让我们尝试一些文件对象属性,看看变量是否仍然存在并且可以访问:print("Filename is '{}'.".format(f.name))
if f.closed:
print("File is closed.")
else:
print("File isn't closed.")
Filename is 'zen_of_python.txt'.
File is closed.
f.read()
---------------------------------------------------------------------------
ValueError Traceback (most recent call last)
~\AppData\Local\Temp/ipykernel_9828/3059900045.py in <module>
----> 1 f.read()
ValueError: I/O operation on closed file.

Python 中的文件读取模式
'r' 打开一个只读文件
'w' 打开一个文件进行写入。如果文件存在,会覆盖它,否则会创建一个新文件
'a' 打开一个仅用于追加的文件。如果该文件不存在,会创建该文件
'x' 创建一个新文件。如果文件存在,则失败
'+' 打开一个文件进行更新
with open('dataquest_logo.png', 'rb') as rf:
with open('data_quest_logo_copy.png', 'wb') as wf:
for b in rf:
wf.write(b)

读取文本文件
read()
方法读取文件的全部内容。如果我们只想从文本文件中读取几个字节怎么办,可以在read()
方法中指定字节数。让我们尝试一下:with open('zen_of_python.txt') as f:
print(f.read(17))
The Zen of Python
with open('zen_of_python.txt') as f:
print(f.readline())
The Zen of Python, by Tim Peters
with open('zen_of_python.txt') as f:
print(f.readline())
print(f.readline())
print(f.readline())
print(f.readline())
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
readline()
方法到达文件末尾时,它返回一个空字符串。with open('zen_of_python.txt') as f:
line = f.readline()
while line:
print(line, end='')
line = f.readline()
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
while
循环之外读取文件的第一行并将其分配给 line
变量。在while
循环中,它打印存储在line
变量中的字符串,然后读取文件的下一行。while
循环迭代该过程,直到readline()
方法返回一个空字符串。空字符串在while
循环中的计算结果为False
,因此迭代过程终止readlines()
方法,将此方法应用于文件对象会返回包含文件每一行的字符串列表with open('zen_of_python.txt') as f:
lines = f.readlines()
print(type(lines))
print(lines)
<class 'list'>
['The Zen of Python, by Tim Peters\n', '\n', 'Beaut...]
print(lines)
print(lines[3:5])
print(lines[-1])
['The Zen of Python, by Tim Peters\n', '\n', 'Beautiful is better than ugly.\n', ... -- let's do more of those!"]
['Explicit is better than implicit.\n', 'Simple is better than complex.\n']
Namespaces are one honking great idea -- let's do more of those!

读取 CSV 文件
import csv
with open('chocolate.csv') as f:
reader = csv.reader(f, delimiter=',')
for row in reader:
print(row)
['Company', 'Bean Origin or Bar Name', 'REF', 'Review Date', 'Cocoa Percent', 'Company Location', 'Rating', 'Bean Type', 'Country of Origin']
['A. Morin', 'Agua Grande', '1876', '2016', '63%', 'France', '3.75', 'Â\xa0', 'Sao Tome']
['A. Morin', 'Kpime', '1676', '2015', '70%', 'France', '2.75', 'Â\xa0', 'Togo']
['A. Morin', 'Atsane', '1676', '2015', '70%', 'France', '3', 'Â\xa0', 'Togo']
['A. Morin', 'Akata', '1680', '2015', '70%', 'France', '3.5', 'Â\xa0', 'Togo']
...
import csv
with open('chocolate.csv') as f:
reader = csv.reader(f, delimiter=',')
for row in reader:
print("The {} company is located in {}.".format(row[0], row[5]))
The Company company is located in Company Location.
The A. Morin company is located in France.
The A. Morin company is located in France.
The A. Morin company is located in France.
The A. Morin company is located in France.
The Acalli company is located in U.S.A..
The Acalli company is located in U.S.A..
The Adi company is located in Fiji.
...
reader()
方法,而是使用返回字典对象集合的 DictReader()
方法。import csv
with open('chocolate.csv') as f:
dict_reader = csv.DictReader(f, delimiter=',')
for row in dict_reader:
print("The {} company is located in {}.".format(row['Company'], row['Company Location']))
The A. Morin company is located in France.
The A. Morin company is located in France.
The A. Morin company is located in France.
The A. Morin company is located in France.
The Acalli company is located in U.S.A..
The Acalli company is located in U.S.A..
The Adi company is located in Fiji.
...

读取 JSON 文件
with
上下文管理器中,我们使用了属于 json 对象的load()
方法,它加载文件的内容并将其作为字典存储在上下文变量中。import json
with open('movie.json') as f:
content = json.load(f)
print(content)
{'Title': 'Bicentennial Man', 'Release Date': 'Dec 17 1999', 'MPAA Rating': 'PG', 'Running Time min': 132, 'Distributor': 'Walt Disney Pictures', 'Source': 'Based on Book/Short Story', 'Major Genre': 'Drama', 'Creative Type': 'Science Fiction', 'Director': 'Chris Columbus', 'Rotten Tomatoes Rating': 38, 'IMDB Rating': 6.4, 'IMDB Votes': 28827}
print(type(content))
<class 'dict'>
print('{} directed by {}'.format(content['Title'], content['Director']))
Bicentennial Man directed by Chris Columbus

总结
open()
内置函数、with
上下文管理器,以及如何读取文本、CSV 和 JSON 等常见文件类型。
CSDN音视频技术开发者在线调研正式上线!
现邀开发者们扫码在线调研

分享
点收藏
点点赞
点在看
关注公众号:拾黑(shiheibook)了解更多
[广告]赞助链接:
四季很好,只要有你,文娱排行榜:https://www.yaopaiming.com/
让资讯触达的更精准有趣:https://www.0xu.cn/
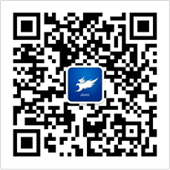
随时掌握互联网精彩
赞助链接
排名
热点
搜索指数
- 1 人民至上 中国式现代化的出发点 7923531
- 2 DeepSeek回答现在能不能入手黄金 7910904
- 3 呼和浩特:三孩可在全市自由择校 7829950
- 4 “投资于人”释放什么信号 7733106
- 5 戚薇秒接台湾朋友的话:也是中国人 7645782
- 6 7800元一根的玉米到底是谁在买 7566871
- 7 男子造谣顶流明星澳门输10亿被拘 7440818
- 8 特朗普大楼遭袭 警方称98人被捕 7393590
- 9 A股总市值逼近100万亿创历史新高 7218921
- 10 今天数学浓度太高了 7182031